Responsive design has become a necessity. This can be problematic, especially using marketo's drag and drop template system. There's a simple approach to overcoming this using jquery.
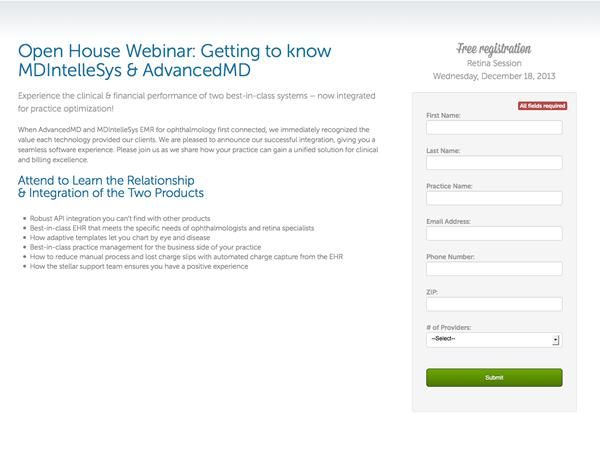
Here's what I've done:
Step 1. Create your responsive layout/template. Be sure to plan a container to hold the marketo form.
<div class="large-input
well"></div>
Step 2. add jquery to your layout. Something like this.
<script src="http://info.advancedmd.com/rs/advancedmd/images/jquery.js"></script>
Step 3. Add the a script to clone the marketo form and place it in your container. Remove the original form.
<script>
/* -- Marketo form alterations by Jeff Petersen -- */
var p = $( ".formwrap .well" ); // create a var that can be referenced later
var f = $( "div.Form_1" ); // var to be used later
var f2 = $( "div.lpeCElement" ); // var for styling
$(document).ready(function(e) {
$( f ).clone().appendTo( p );
var form_id = $( f ).attr("id");
$(f).remove();
}); </script>
Step 4. Add classes and styles to make the marketo form match your styling. These should match what your design is expecting.
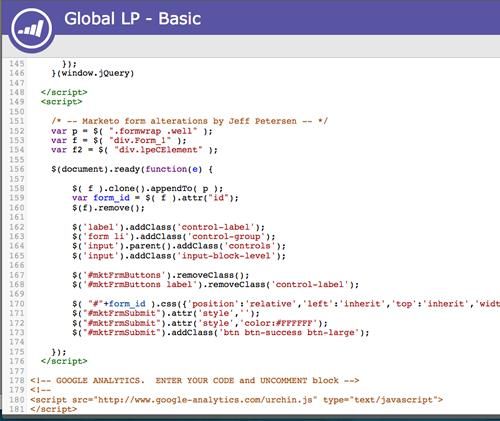
...$(document).ready(function(e) {
$( f ).clone().appendTo( p );
var form_id = $( f ).attr("id");
$(f).remove();
$( "#"+form_id ).css({'position':'relative','left':'inherit','top':'inherit','width':'100%'}); //this resets the forms native positioning to use local or relative positioning instead of absolute //These styles are specific to my page. Your's may be similar.
//They override default styles and add new classes to control the style
$('label').addClass('control-label');
$('form li').addClass('control-group');
$('input').parent().addClass('controls');
$('input').addClass('input-block-level');
$('#mktFrmButtons').removeClass();
$('#mktFrmButtons label').removeClass('control-label');
$("#mktFrmSubmit").attr('style',''); //remove default marketo button style
$("#mktFrmSubmit").attr('style','color:#FFFFFF');
$("#mktFrmSubmit").addClass('btn btn-success btn-large'); });
</script>...
Step 5. Create a new landing page using your new template. Using the editor drag a form onto the lp design. Set it up as usual. Approve the draft. Preview should now show the form in your new container.
Marketo will now track traffic and conversion based on form completion. It also allows you to use any form without altering the forms code.